In part 1 of this series, I was searching for a device that is easy to use for seniors. More specifically, a device easy to use for people who have some form of dementia. What I found is that a lot of the devices already on the market have too many options. This makes the device more difficult to use and confusing for the user. Finding a suitable application to fit what I was looking for was tough leading me to build my own hardware and application.
There are applications already present in the market that work great or have great features e.g. calling, news, music, games. The problem with most of these options, from my perspective, is that there are too many buttons. I needed, err my father needed a simpler User Interface and User Experience.
Requirements
So, what are the requirements I was trying to meet?
- Must Have Features
- News
- Music
- Family pictures
- Simplified User Interface
- Minimal buttons
- Modern look
- Easy to use
First Attempt
The search began to find the best way to build an application on Raspbian that would meet my requirements. I will add in that I was looking for a moderately quick way to build out this application which is when I found: Xojo: Cross-platform development tool.
I quickly began to learn Xojo's object-oriented language but eventually hit a wall. Moreover, it was seeming to be a fair amount of code for not a lot of gain. I decided to abandon Xojo as it came down to the fact that the HTMLViewer was hardly responsive. Here is a quick glimpse at the app I wrote using Xojo... I had to find another solution though.
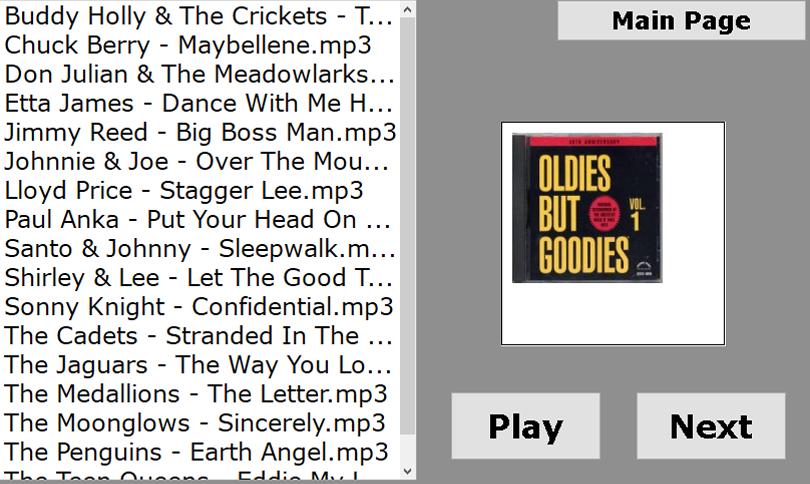
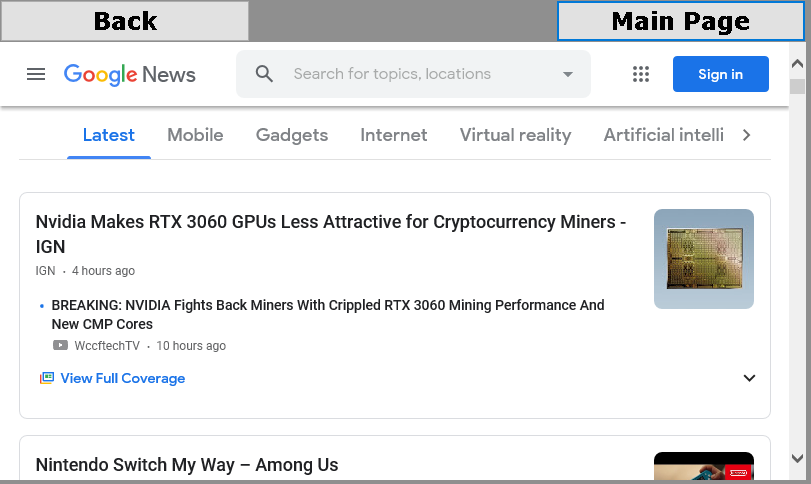
This is when I began down the path of a web application built on PHP, HTML5, CSS3, Javascript, and jQuery. Going this route was fun, as I was able to pick up quite a bit of coding along the way.
The app is built into three key parts - Home, News, and Music.
Clock/Picture Frame (Home Page)
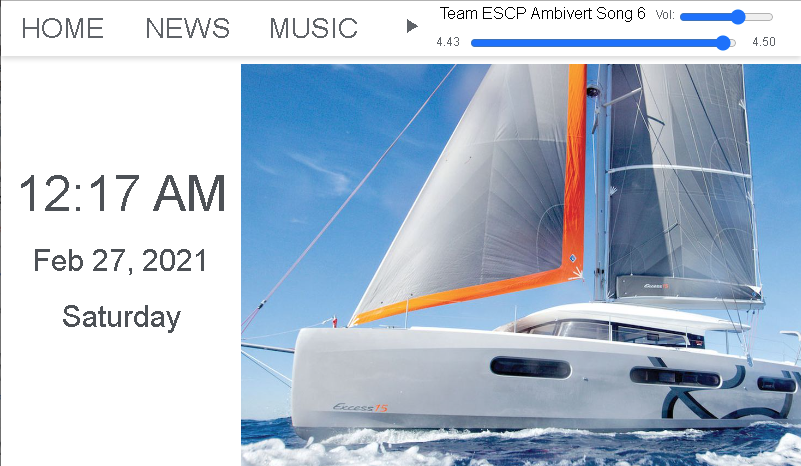
Here's a breakdown of how the main page loads all three sub-pages (home, news, music) upon opening the site. This ensures that all of the data is loaded in the background and can be quickly accessed like a desktop application. The audio player in the top right corner is also loaded upon page load, we'll talk about that in another part of this series.
Main Page Layout
<div id="navbar">
<ul id="navbarul">
<li><a data-target="main">HOME</a></li>
<li><a data-target="news">NEWS</a></li>
<li><a data-target="music">MUSIC</a></li>
</ul>
<div id="audio"></div>
</div>
<div id="content">
<div id="main" class="content"></div>
<div id="news" class="content"></div>
<div id="music" class="content"></div>
</div>
To easily load the elements of the page all at once and quickly switch tabs, we use jQuery! Why use jQuery to load the elements? Because, the jQuery $(document).ready()
does a lot for us, checking when the DOM is ready. If we did this in plain Javascript, it would require a bit more code; the trade-off is loading the jQuery library, given its only about 32KB. A good read on this was posted by Jan Kyu Peblik - https://stackoverflow.com/a/9899701
Limitations... cross-browser compatibility.
$(document).ready(function () {
$('#main').load('main.php');
$('#news').load('news.php').hide();
$('#music').load('music.php').hide();
}
$(function () {
$('#navbar ul li a').click(function () {
var page = $(this).attr('data-target');
$('.content').hide();
$('#' + page).show();
return false;
});
});
});
After the pages are loaded, we use the .click()
method on our menu. So we do a few things when there is a click - we store the element name in the data-target
attribute of that link, we hide the elements with the class name content
, and we show the previously stored element from the data-target
attribute. Using the data-target attribute, as opposed to the id attribute ensures it does not hide our menu item when showing or hiding an element! Finally, return false stops the default action of that event!
Fun fact... when using data-anything attributes in html we can store extra information without affecting the element. https://developer.mozilla.org/en-US/docs/Learn/HTML/Howto/Use_data_attributes
In the next part of this series, we'll look at using rss feeds to build an easy to read news page and HTML5 audio player!